@adhamu/react-search-suggestions 中文文档教程
React Search Suggestions
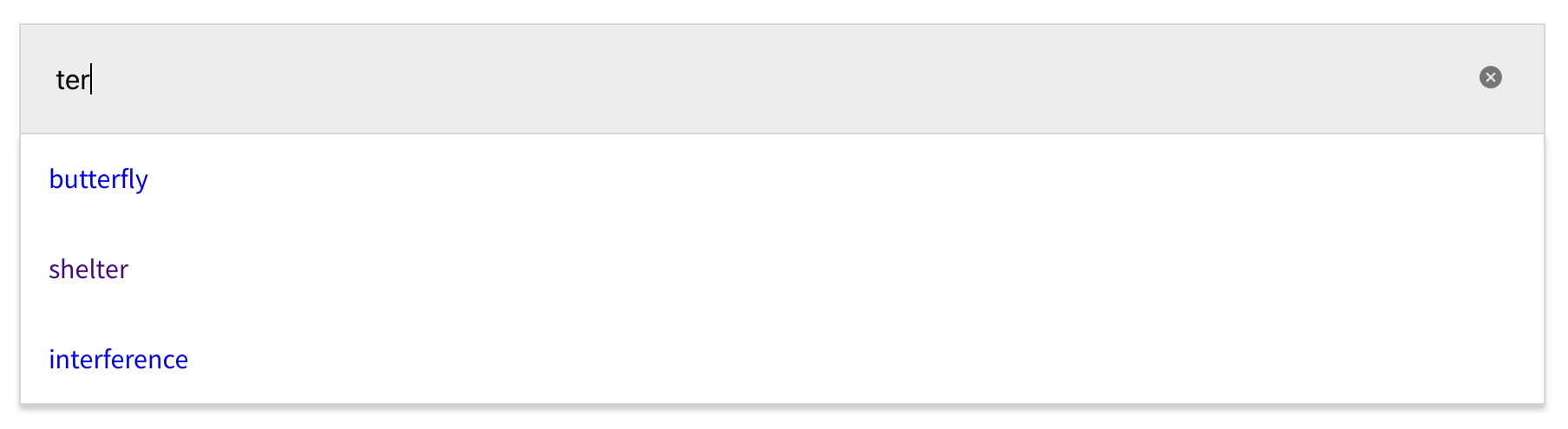
Prerequisities
- React (obviously)
Installation
yarn add @adhamu/react-search-suggestions
Usage
import React from 'react'
import { SearchSuggestions } from '@adhamu/react-search-suggestions'
const MyComponent = () => (
<SearchSuggestions
autoFocus={true}
suggestions={[
'polite',
'fastidious',
'dull',
'pudding',
'mole',
'angle',
].map(word => (
<a href={`https://www.google.co.uk/search?q=${word}`}>{word}</a>
))}
/>
)
export default MyComponent
HTML Structure
标记非常简单。 您带来填充每个搜索建议的内容。 在这个例子中:
<div>
<input />
<ul>
<li>
<a href="https://www.google.co.uk/search?q=polite">polite</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=fastidious">fastidious</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=dull">dull</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=pudding">pudding</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=mole">mole</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=angle">angle</a>
</li>
</ul>
</div>
Props
Prop | Description | Type | Default | Required? |
---|---|---|---|---|
suggestions | A collection of HTML elements or React components used for search suggestions | React.ReactNode[] | Y | |
id | ID for entire component | string | undefined | N |
className | Optional class name to style component | string | '' | N |
name | Input name | string | 'q' | N |
placeholder | Input placeholder | string | 'Search' | N |
autoFocus | Input autoFocus | boolean | false | N |
onChange | Input onChange handler | function | undefined | N |
withStyles | Basic styling for the component | boolean | false | N |
Styling
默认情况下,组件几乎没有任何样式。 鉴于标记的语义性质,很容易用 CSS 定位这些标记。 如上所述,您可以为此向组件提供一个 className
。
或者,您可以将 withStyles
属性设置为 true
以实现一些非常基本的样式。 在 GitHub 页面 上可以看到这方面的示例。
重要:每个顶级元素的搜索建议中的:focus
属性决定了所选元素的活动状态。 请参阅上面的 HTML 结构 以正确确定任何 CSS 选择器。
Arrow Key Navigation
您可以免费获得此功能,这也是创建此共享组件的主要动机。
React Search Suggestions
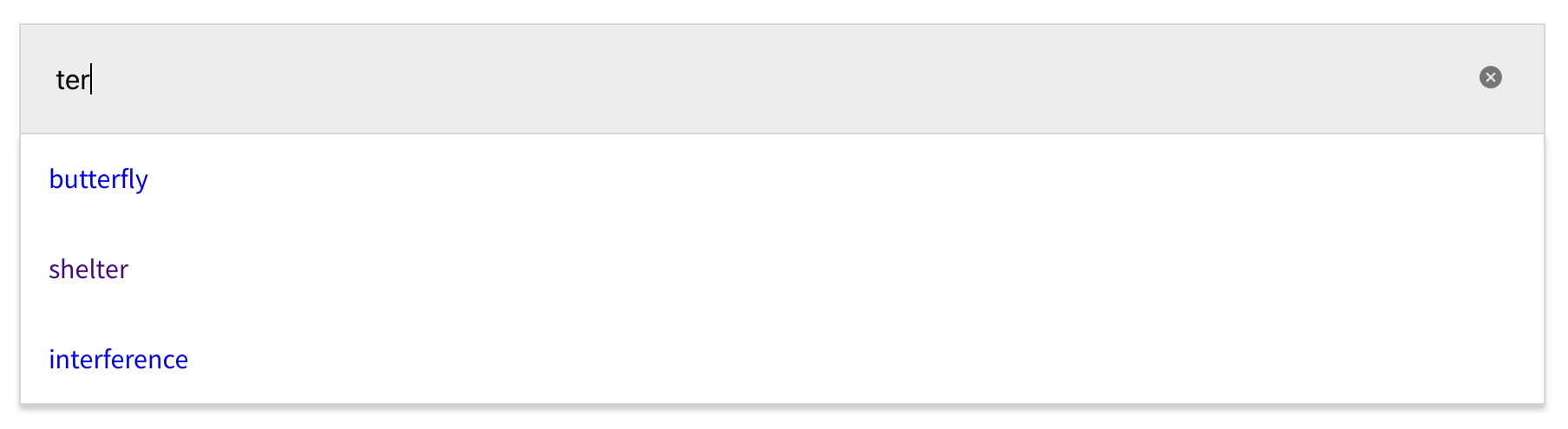
Prerequisities
- React (obviously)
Installation
yarn add @adhamu/react-search-suggestions
Usage
import React from 'react'
import { SearchSuggestions } from '@adhamu/react-search-suggestions'
const MyComponent = () => (
<SearchSuggestions
autoFocus={true}
suggestions={[
'polite',
'fastidious',
'dull',
'pudding',
'mole',
'angle',
].map(word => (
<a href={`https://www.google.co.uk/search?q=${word}`}>{word}</a>
))}
/>
)
export default MyComponent
HTML Structure
The markup is very simple. You bring what populates each search suggestion. In this example:
<div>
<input />
<ul>
<li>
<a href="https://www.google.co.uk/search?q=polite">polite</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=fastidious">fastidious</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=dull">dull</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=pudding">pudding</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=mole">mole</a>
</li>
<li>
<a href="https://www.google.co.uk/search?q=angle">angle</a>
</li>
</ul>
</div>
Props
Prop | Description | Type | Default | Required? |
---|---|---|---|---|
suggestions | A collection of HTML elements or React components used for search suggestions | React.ReactNode[] | Y | |
id | ID for entire component | string | undefined | N |
className | Optional class name to style component | string | '' | N |
name | Input name | string | 'q' | N |
placeholder | Input placeholder | string | 'Search' | N |
autoFocus | Input autoFocus | boolean | false | N |
onChange | Input onChange handler | function | undefined | N |
withStyles | Basic styling for the component | boolean | false | N |
Styling
By default, the component comes with almost no styles. Given the semantic nature of the markup, it is quite easy to target these with CSS. As mentioned above, you can provide a className
to the component for this.
Alternatively, you can set the withStyles
prop to true
to achieve some very basic styling. An example of this can be seen on GitHub Pages.
Important: The :focus
attribute on each top level element's search suggestion is what powers the active state of a selected element. Refer to the HTML Structure above to correctly determine any CSS selectors.
Arrow Key Navigation
You get this functionality for free and was the main motivation for creating this shared component.
你可能也喜欢
- @0xcert/merkle 中文文档教程
- @0xtosha/blockchain-addressbook 中文文档教程
- @2o3t/electron-ipc-promise 中文文档教程
- @36node-lecha/locate-sdk-js 中文文档教程
- @3liv/rijs.sync 中文文档教程
- @a11ywatch/website-source-builder 中文文档教程
- @aaronjan/create-typescript-project 中文文档教程
- @ableneo/prettier-config 中文文档教程
- @aboutweb/proxyscope 中文文档教程
- @abradley2/redux-loop 中文文档教程