@adactiveasia/adsm-steplist 中文文档教程
Step List
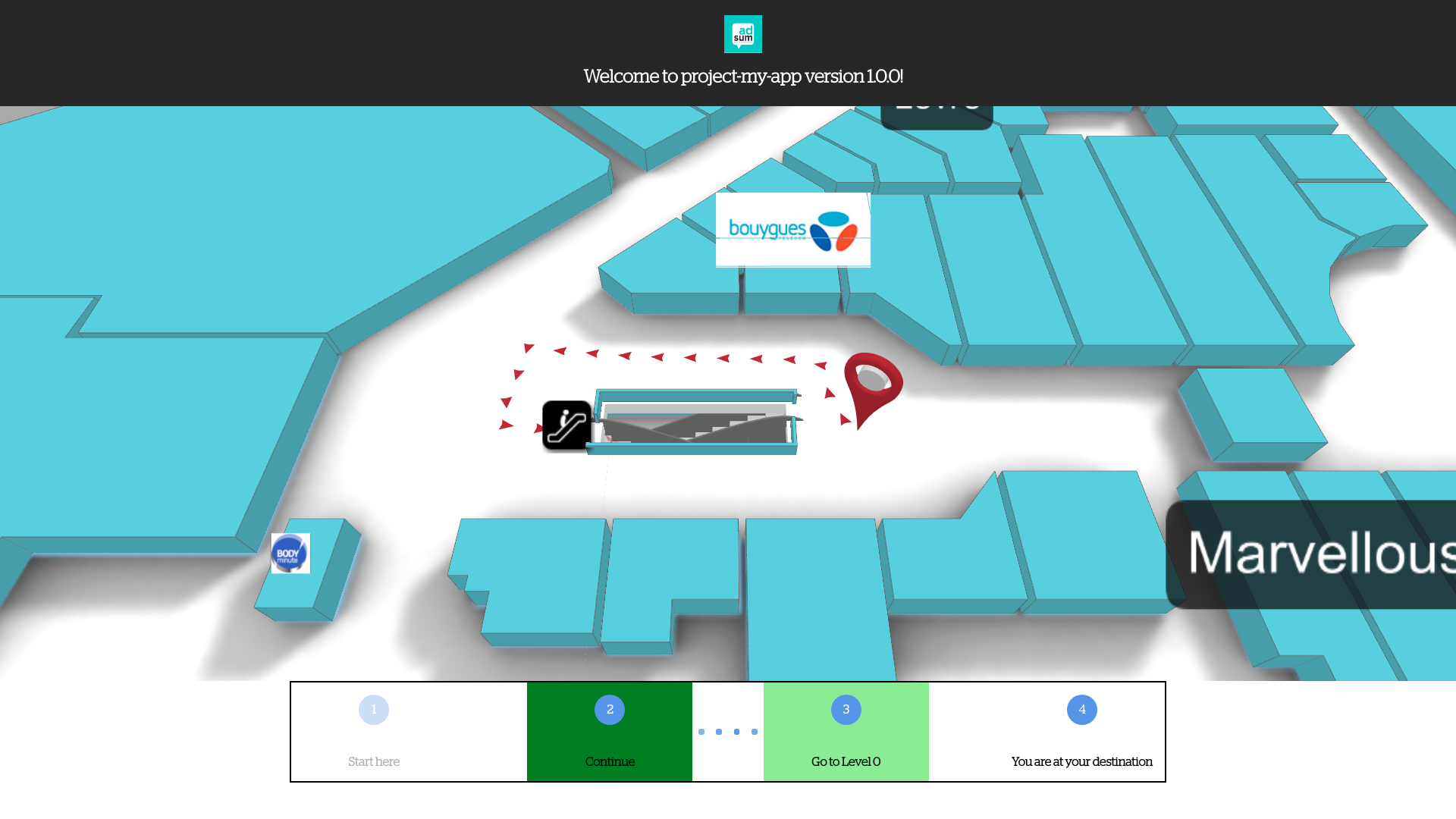
Getting started
npm i --save @adactive/arc-steplist
或者
yarn add @adactive/arc-steplist
How to use
只需添加如下组件:
import * as React from 'react';
// import StepList component
import StepList from '@adactive/arc-steplist';
/*...*/
class MyComponent extends React.Component {
render() {
return (
<StepList placeId={placeId} />
);
}
}
Props
type OwnPropsType = {|
placeId: ?number,
pmr: boolean, // optional
messages: MessagesType, // optional
stepStyle: StepStyleType, // optional
renderStep: RenderStepType, // optional
renderStepTail: RenderStepTailType, // optional
|};
您还可以在此处看到导出的流类型。 您可以将它们从组件导入到 增强您在应用程序中的流程输入。
export type StepType = {|
index: number,
floor: ?{|
id: number,
name: ?string,
deltaAltitudeWithPrevStep: number,
|},
message: string,
|};
export type MessagesType = (step: StepType) => {|
firstStep?: string,
lastStep?: string,
isInterfloor?: string,
default?: string,
|};
export type StepStyleType = {|
default?: { [key: string]: string},
isDone?: { [key: string]: string},
current?: { [key: string]: string},
isNext?: { [key: string]: string},
isNotDoneYet?: { [key: string]: string},
|};
export type RenderStepType = (
mode: StepModeType,
step: StepType,
stepStyle: StepStyleType,
onClick: (stepIndex: number) => () => void,
) => ?Node;
export type RenderStepTailType = (mode: StepModeType, step: StepType) => ?Node;
placeId
步骤列表定位的地点的地点 ID。 这是组件唯一必要的道具。
pmr
是否使用 PMR 绘制寻路(避开楼梯,改用电梯)。
default value
defaultPmr = false
messages
自定义每个步骤上显示的消息,遵循此结构:
type MessagesType = (step: StepType) => {|
firstStep?: string,
lastStep?: string,
isInterfloor?: string,
default?: string,
|};
- firstStep: the first step.
- lastStep: the last step.
- isInterfloor: steps where the floor is different from the previous one.
- default: message for steps that don't fall into any of the previous categories.
default value
defaultMessages = (step: StepType) => {
const { floor } = step;
let floorName = null;
let upOrDown = '';
if (floor) {
const { name, deltaAltitudeWithPrevStep } = floor;
// floor name
floorName = name;
// interfloor direction
if (deltaAltitudeWithPrevStep > 0) upOrDown = ' up'; // space before on purpose
else if (deltaAltitudeWithPrevStep < 0) upOrDown = ' down'; // same
}
return {
firstStep: `Start here${floorName ? `, at ${floorName}` : ''}`,
lastStep: 'You are at your destination',
isInterfloor: floorName ? `Go${upOrDown} to ${floorName}` : 'Change floor',
default: 'Continue',
};
}
stepStyle
自定义每个步骤的样式,遵循此结构:
export type StepStyleType = {|
default?: CSSStyleDeclaration,
isDone?: CSSStyleDeclaration,
current?: CSSStyleDeclaration,
isNext?: CSSStyleDeclaration,
isNotDoneYet?: CSSStyleDeclaration,
|};
- default: style applied to each step, whatever their current mode.
- isDone: style added to step that are done.
- current: style added to current step.
- isNext: style added to the step just after the current step.
- isNotDoneYet: style added to the steps that are after the 'isNext' step, not done yet.
请注意,您不必修改所有样式。 例如,您只能修改 'isNext' 样式,其他默认样式将被保留。
如果要修改整个步骤列表的样式,可以覆盖'steplist' 组件样式表中的类名。
default value
defaultStepStyle = {
default: {
transition: 'all .5s',
},
isDone: {
opacity: 0.3,
},
current: {
backgroundColor: 'green',
},
isNext: {
backgroundColor: 'lightgreen',
},
isNotDoneYet: {},
}
renderStep
覆盖默认渲染步骤函数。 这比仅自定义 带有 stepStyle 属性的样式。
export type RenderStepType = (
mode: StepModeType,
step: StepType,
stepStyle: StepStyleType,
onClick: (stepIndex: number) => () => void,
) => ?Node;
这是一个返回一些 JSX 的函数,以便您能够使用当前模式,步骤, stepStyle 和您自己步骤中的默认点击处理程序。
default value
defaultRenderStep = (
mode: StepModeType,
step: StepType,
stepStyle: StepStyleType,
onClick: (stepIndex: number) => () => void,
) => (
<div
className="step"
style={stepStyle}
onClick={onClick(step.index)}
role="complementary"
onKeyDown={() => {}}
>
<div className="badge">{step.index + 1}</div>
<div className="message">{step.message}</div>
</div>
)
renderStepTail
与 renderStep 相同,但对于步骤尾部:每个步骤之前的空间。
如果您想删除尾巴,只需传递一个返回 null 的函数。
export type RenderStepTailType = (mode: StepModeType, step: StepType) => ?Node;
同样,这是一个函数,因此您可以在自己的步骤尾巴中使用当前模式 和步骤。
default value
defaultRenderStepTail = (mode: StepModeType, step: StepType) => {
if (step.index === 0) return null; // no tail before first step
const numberOfCircles = 4;
// [0, 1, ..., numberOfCircles - 1]
const dumbArrayToMap = [...Array(numberOfCircles).keys()];
const delayBetweenEachCircle = 1 / 10;
// wait for the last circle to finish + add 1 more delay to make it smoother
const animationDuration = (numberOfCircles + 2) * delayBetweenEachCircle;
const circleStyle = delay => (
{
width: `${100 / (numberOfCircles * 3)}%`,
maxWidth: '1em',
animation: mode !== 'isNext' ? null // animation only if step is next
: `blink ${animationDuration}s linear ${delay}s infinite alternate`,
}
);
return (
<div className="tail">
{dumbArrayToMap.map(index => (
<div
// 'index' as key is ok here,
// because no modification will happen on numberOfCircles array
key={index}
className="circle"
style={circleStyle(index * delayBetweenEachCircle)}
/>
))}
</div>
);
}
Copy component inside your project src folder
它会将组件复制到您的项目中,位于 src/components/adsum-steplist/ 中。
npx @adactive/arc-steplist copy
Step List
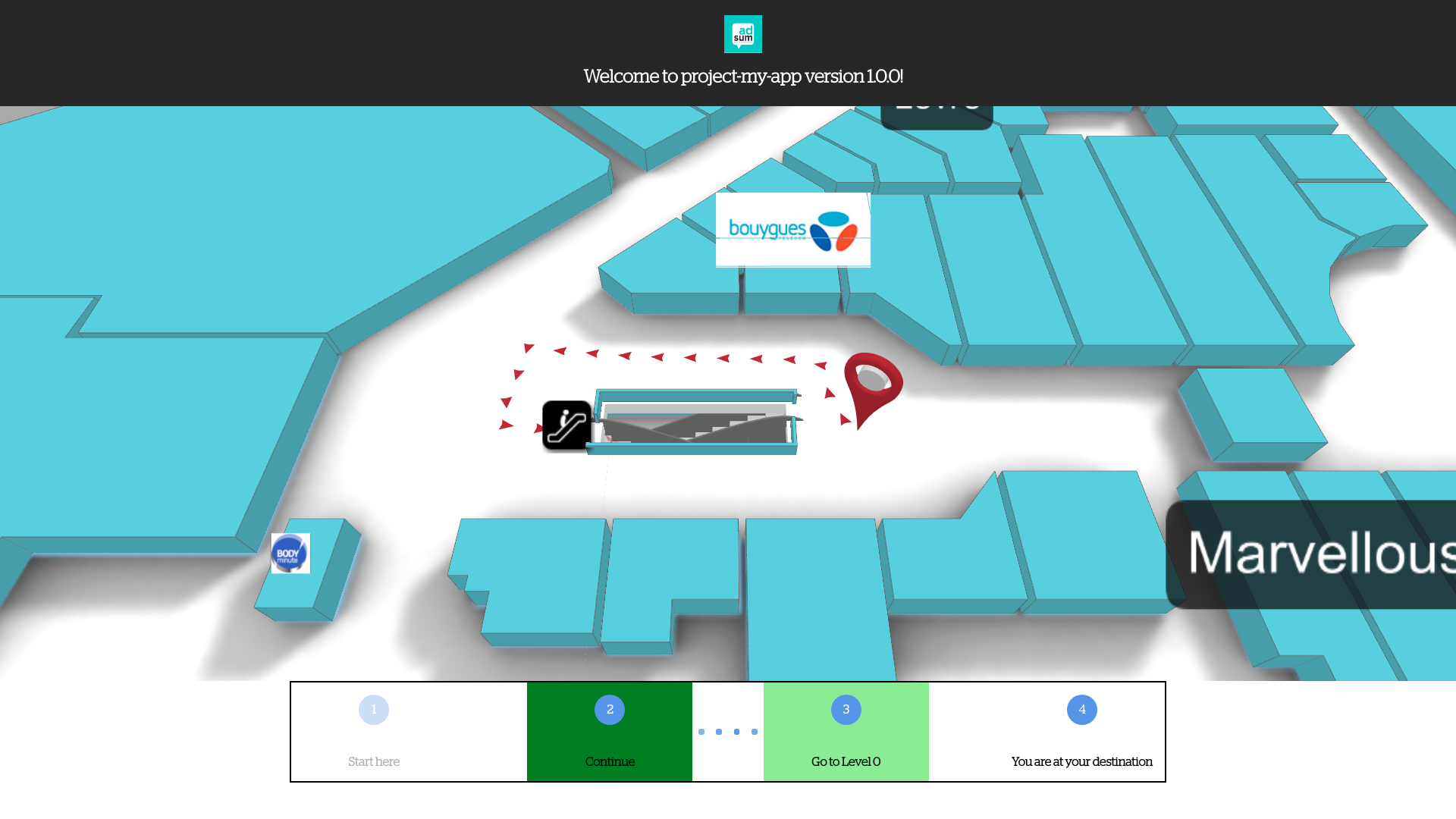
Getting started
npm i --save @adactive/arc-steplist
OR
yarn add @adactive/arc-steplist
How to use
Just add the component as below:
import * as React from 'react';
// import StepList component
import StepList from '@adactive/arc-steplist';
/*...*/
class MyComponent extends React.Component {
render() {
return (
<StepList placeId={placeId} />
);
}
}
Props
type OwnPropsType = {|
placeId: ?number,
pmr: boolean, // optional
messages: MessagesType, // optional
stepStyle: StepStyleType, // optional
renderStep: RenderStepType, // optional
renderStepTail: RenderStepTailType, // optional
|};
You can see here as well what flow types are exported. You can import them from the component to enhance your flow typing in your app.
export type StepType = {|
index: number,
floor: ?{|
id: number,
name: ?string,
deltaAltitudeWithPrevStep: number,
|},
message: string,
|};
export type MessagesType = (step: StepType) => {|
firstStep?: string,
lastStep?: string,
isInterfloor?: string,
default?: string,
|};
export type StepStyleType = {|
default?: { [key: string]: string},
isDone?: { [key: string]: string},
current?: { [key: string]: string},
isNext?: { [key: string]: string},
isNotDoneYet?: { [key: string]: string},
|};
export type RenderStepType = (
mode: StepModeType,
step: StepType,
stepStyle: StepStyleType,
onClick: (stepIndex: number) => () => void,
) => ?Node;
export type RenderStepTailType = (mode: StepModeType, step: StepType) => ?Node;
placeId
Place ID of the place targeted by the steplist. This is the only necessary prop of the component.
pmr
Draw the wayfinding with PMR (avoid stairs, use elevator instead) or not.
default value
defaultPmr = false
messages
Customize message shown on each step, following this structure:
type MessagesType = (step: StepType) => {|
firstStep?: string,
lastStep?: string,
isInterfloor?: string,
default?: string,
|};
- firstStep: the first step.
- lastStep: the last step.
- isInterfloor: steps where the floor is different from the previous one.
- default: message for steps that don't fall into any of the previous categories.
default value
defaultMessages = (step: StepType) => {
const { floor } = step;
let floorName = null;
let upOrDown = '';
if (floor) {
const { name, deltaAltitudeWithPrevStep } = floor;
// floor name
floorName = name;
// interfloor direction
if (deltaAltitudeWithPrevStep > 0) upOrDown = ' up'; // space before on purpose
else if (deltaAltitudeWithPrevStep < 0) upOrDown = ' down'; // same
}
return {
firstStep: `Start here${floorName ? `, at ${floorName}` : ''}`,
lastStep: 'You are at your destination',
isInterfloor: floorName ? `Go${upOrDown} to ${floorName}` : 'Change floor',
default: 'Continue',
};
}
stepStyle
Customize style of each step, following this structure:
export type StepStyleType = {|
default?: CSSStyleDeclaration,
isDone?: CSSStyleDeclaration,
current?: CSSStyleDeclaration,
isNext?: CSSStyleDeclaration,
isNotDoneYet?: CSSStyleDeclaration,
|};
- default: style applied to each step, whatever their current mode.
- isDone: style added to step that are done.
- current: style added to current step.
- isNext: style added to the step just after the current step.
- isNotDoneYet: style added to the steps that are after the 'isNext' step, not done yet.
Be aware that you don't have to modify all the styles. For examples, you can modify only the 'isNext' style, and the others default styles will be kept.
If you want to modify the style of the whole step list, you can overwrite the 'steplist' classname in your component stylesheet.
default value
defaultStepStyle = {
default: {
transition: 'all .5s',
},
isDone: {
opacity: 0.3,
},
current: {
backgroundColor: 'green',
},
isNext: {
backgroundColor: 'lightgreen',
},
isNotDoneYet: {},
}
renderStep
Overwrite the default render step function. This is one step further than just customizing the style with stepStyle prop.
export type RenderStepType = (
mode: StepModeType,
step: StepType,
stepStyle: StepStyleType,
onClick: (stepIndex: number) => () => void,
) => ?Node;
This is a function that return some JSX,for you to be able to use the current mode, the step, the stepStyle and the default click handler in your own steps.
default value
defaultRenderStep = (
mode: StepModeType,
step: StepType,
stepStyle: StepStyleType,
onClick: (stepIndex: number) => () => void,
) => (
<div
className="step"
style={stepStyle}
onClick={onClick(step.index)}
role="complementary"
onKeyDown={() => {}}
>
<div className="badge">{step.index + 1}</div>
<div className="message">{step.message}</div>
</div>
)
renderStepTail
Same as renderStep, but for the step tail: the space before each step.
If you want to remove the tails, simply pass a function that returns null.
export type RenderStepTailType = (mode: StepModeType, step: StepType) => ?Node;
Again, this a function, so you can use the current mode and the step in your own step tails.
default value
defaultRenderStepTail = (mode: StepModeType, step: StepType) => {
if (step.index === 0) return null; // no tail before first step
const numberOfCircles = 4;
// [0, 1, ..., numberOfCircles - 1]
const dumbArrayToMap = [...Array(numberOfCircles).keys()];
const delayBetweenEachCircle = 1 / 10;
// wait for the last circle to finish + add 1 more delay to make it smoother
const animationDuration = (numberOfCircles + 2) * delayBetweenEachCircle;
const circleStyle = delay => (
{
width: `${100 / (numberOfCircles * 3)}%`,
maxWidth: '1em',
animation: mode !== 'isNext' ? null // animation only if step is next
: `blink ${animationDuration}s linear ${delay}s infinite alternate`,
}
);
return (
<div className="tail">
{dumbArrayToMap.map(index => (
<div
// 'index' as key is ok here,
// because no modification will happen on numberOfCircles array
key={index}
className="circle"
style={circleStyle(index * delayBetweenEachCircle)}
/>
))}
</div>
);
}
Copy component inside your project src folder
It will copy the component inside your project, in src/components/adsum-steplist/.
npx @adactive/arc-steplist copy