@2600hz/sds-react-native-components 中文文档教程
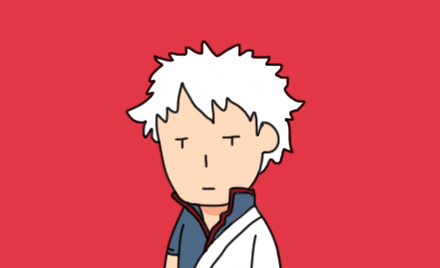
@2600hz/sds-react-native-components
包含用于所有 React 本机应用程序开发的所有可共享组件的库。
[](https://img.shields.io/ npm/v/@2600hz/sds-react-native-components?style=flat-square) [](https://github.com/prettier/prettier)
2600Hz - Commio
SDS React Native Components Library
Installation
yarn add @2600hz/sds-react-native-components
Needed packages
如果您的应用中还没有它们,yarn 将安装可用性需要以下软件包。 这些包被添加为 peerDependencies
- @2600hz/commio-native-utilities - Contains useful js functions and shareable configurations
- @2600hz/sds-react-native-theme - Contains theme available for react native apps that use styled-components
- Styled-Components - Library to style react components
- react-native-svg - Provides SVG support to React Native on iOS and Android, and a compatibility layer for the web
- tslib - Contains all of the TypeScript helper functions. Even if your project doesn't use Typescript, you need this package for native-components to function properly
Packages considerations
如果您的应用程序中已有这些库,则需要对配置文件进行以下更改才能使包正常运行 在您的 App 根文件夹上执行此操作
// metro.config.js
const modules = [
'@2600hz/commio-native-utilities',
'@2600hz/sds-react-native-theme',
'react',
'react-dom',
'react-native',
'react-native-svg',
'styled-components',
'tslib',
];
module.exports = (async () => {
return {
// ...
resolver: {
extraNodeModules: modules.reduce((acc, name) => {
acc[name] = path.join(__dirname, 'node_modules', name);
return acc;
}, {}),
},
// ...
};
})();
现在在 webpack
文件中使用它
// webpack.config.js
const metroConfig = require('./metro.config');
const node_modules = path.join(__dirname, 'node_modules');
module.exports = async function (env, argv) {
const config = await createExpoWebpackConfigAsync(env, argv);
const { resolver } = await metroConfig;
// We need to make sure that only one version is loaded for peerDependencies
// So we alias them to the versions in example's node_modules
Object.assign(config.resolve.alias, {
...resolver.extraNodeModules,
});
return config;
};
Styled-Components considerations 我们使用为 styled-components 构建的主题。 样式化组件可以从两个来源使用
- The one installed automatically when installing @2600hz/sds-react-native-components
- The one installed manually by you on your app
在第一种情况下,组件包将使用您在 ThemeProvider 上设置的主题,用于您自己的组件和包中的内置组件。 如果你在你的应用程序上安装 styled-components,组件包将不会使用你自动设置的主题。 因此,您需要确保只安装一个样式组件,即应用程序。
我们通过对之前显示的 metro-config.js 和 webpack-config.js 进行更改来解决此问题。
React Native Svg 注意事项 应该只安装一个版本的 react-native-svg,如果您在应用中手动安装它,请确保将其添加到 extraNodeModules
列表中,以便我们确保只使用一个版本。 否则它会在执行时抛出错误。
Usage
它依赖于 styled-components,因此它需要为应用程序设置一个主题。 从 @2600hz/sds-react-native-theme 获取主题 您可以将 DefaultTheme 设置为来自 sds-react-native-theme
的那个。 创建一个声明文件,将其命名为约定 styled.d.ts
import {} from 'styled-components';
import { DefaultThemeProps } from '@2600hz/sds-react-native-theme';
declare module 'styled-components' {
export interface DefaultTheme extends DefaultThemeProps {}
}
现在在你的入口点组件中使用它
import { lightTheme } from '@2600hz/sds-react-native-theme';
<ThemeProvider theme={lightTheme}>
{/* <App ... /> */}
{children}
</ThemeProvider>
这个主题将适用于你的组件和来自 sds-react-native-components 的组件
现在在你的组件
import { Button, Telicon } from "@2600hz/sds-react-native-components";
// ...
<Button onPress={handleCall} title="Call" color="#aaffcc" />
<View>
<Telicon
name="volume-x"
size="xsmall"
fill="green"
fillSecondary="pink"
/>
</View>
Svg Files
包已经有组件来处理 svg xml/文件。 这些是
- SvgFromXml - Renders an icon, it accepts a svg xml string or file (Internally calls the following two)
- SvgFromXmlString - Renders an icon, it accepts a svg xml string
- SvgFromXmlFile - Renders an icon, it accepts a svg xml file
例子
const xml = `<svg viewBox="0 0 512 512"> <path d="M472.916..." /></svg>`;
<SvgFromXml xml={xml} size="small" fill="green" />
<SvgFromXmlString xml={xml} size="medium" fill="blue" />
// ...
import UserIcon from './app/assets/user.svg';
<SvgFromXml xml={UserIcon} size="xsmall" fill="yellow" />
<SvgFromXmlFile Xml={UserIcon} size="xlarge" fill="magenta" />
如果你想自己处理 svg 文件或需要一个不包含在 telicon 中的不同图标,然后创建一个 declarations.d.ts
(如果你已经有一个,然后以不同的方式命名) 将此内容添加到文件
declare module '*.svg' {
import { SvgXmlFileType } from '@2600hz/sds-react-native-components';
const content: SvgXmlFileType;
export default content;
}
然后你可以通过这种方式导入svg文件
import UserIcon from './app/assets/user.svg';
<UserIcon width={100} height={100} fill="red" />
使用@2600hz/commio-native-utilities提供的svgrrc配置来处理svgs的转换方式
const { svgrrcBaseConfig } = require('@2600hz/commio-native-utilities');
module.exports = {
...svgrrcBaseConfig,
native: true,
};
我们使用svgo 配置 svg xml 应如何转换为 JSX。 寻找可用的插件,这样您就可以决定应该移动哪些属性。 例如,我们希望尽可能将所有颜色转换为字符串(例如 #f00 为红色)
const { svgrrcBaseConfig } = require('@2600hz/commio-native-utilities');
module.exports = {
...svgrrcBaseConfig,
native: true,
svgoConfig: {
plugins: [
{ convertColors: true },
...
]
}
};
Development
此项目需要 Node.js v14+ 才能运行.
使用 .nvmrc 文件有助于规范所有维护者使用的节点版本。 如果您需要使用此文件中指定的版本,请运行这些命令。
nvm use
nvm install
使用包管理器 yarn v1+ 安装依赖项和 devDependencies。
yarn install
yarn
创建符号链接 在包根文件夹上运行以下命令,以便您可以在本地使用应用
yarn link
观看项目 每当有变化时构建包
yarn watch
使用包 两个项目必须放在同一个文件夹中,否则将无法工作
yarn link @2600hz/sds-react-native-components
安装包的注意事项
- Add package as alias in
babel.config.js
// babel.config.js
const path = require('path');
module.exports = function (api) {
api.cache(true);
return {
// ...
plugins: [
// ...
[
'module-resolver',
{
alias: {
// ...
// For development, we want to alias the library to the source
// Remember we have the package folder right next to the app
'@2600hz/sds-react-native-components': path.join(__dirname, '..', 'sds-react-native-components', 'src/index'),
},
},
],
],
};
};
- Blacklist peerDependencies to ensure only one version of packages is used (The one from App)
// metro.config.js
const path = require('path');
const blacklist = require('metro-config/src/defaults/blacklist');
const escape = require('escape-string-regexp');
// Assuming your package folder is named "sds-react-native-components" and it's located right next to the app folder
const root = path.resolve(__dirname, '..', 'sds-react-native-components');
const modules = [
'@2600hz/commio-native-utilities',
'@2600hz/sds-react-native-theme',
'react',
'react-dom',
'react-native',
'react-native-svg',
'styled-components',
'tslib',
];
module.exports = (async () => {
return {
projectRoot: __dirname,
watchFolders: [root],
resolver: {
// We need to make sure that only one version is loaded for peerDependencies
// So we blacklist them at the root, and alias them to the versions in example's node_modules
blacklistRE: blacklist(
modules.map(
(m) =>
new RegExp(`^${escape(path.join(root, 'node_modules', m))}\\/.*$`)
)
),
extraNodeModules: modules.reduce((acc, name) => {
acc[name] = path.join(__dirname, 'node_modules', name);
return acc;
}, {}),
},
};
})();
@2600hz/sds-telicon 此包包含 telicon svg 的 xml 定义,我们提供 SvgTelicon
和 Telicon
组件,以便应用程序轻松在屏幕上呈现图标。 每次 sds-telicon 更新时,我们需要在我们的组件包上更新它的版本,自动将 Telicon 组件更新为新的 telicon.svg 更改。 如果要手动将 telicon.svg 转换为组件运行
yarn svg:jsx
注意:每次“yarn install”运行时都会执行此命令
构建包 将自动运行 bob build
yarn build
Utilities
使用 Eslint
yarn run lint:fix
格式化代码使用 Prettier
yarn run format
yarn run prettify
检查文件上的 Typescript
yarn run typescript
运行完整检查
yarn run full-check
Tech
组件库使用了一些开源项目正常工作:
- React.js - JavaScript library for building user interfaces.
- React Native - JavaScript library for creating native apps for Android and IOS using React.
- Typescript - Strongly typed programming language which builds on JavaScript
- NodeJs - Allows execute javascript scripts on the terminal
- Storybook - Development environment for UI components
- Luxon - library for dealing with dates and times in JavaScript (better alternative than Moment.js)
- Styled-Components - Library to style react components
- react-native-svg - Provides SVG support to React Native on iOS and Android, and a compatibility layer for the web
- create-react-native-library - CLI to scaffold React Native libraries
- @2600hz/sds-react-native-theme - React Native Theme for Styled-components
- @2600hz/commio-native-utilities - Library written in js for shareable config files and common functions
Contributing
欢迎请求请求。 对于重大更改,请先打开一个问题来讨论您想要更改的内容。
请参阅贡献指南,了解如何为存储库和开发工作流程做出贡献。
承诺 提交必须遵循常规提交格式 确保您的消息看起来像以下示例
feat: Adding new badge component
fix: Touchable component not being exported correctly
fix!: Drop support for Typescript
请注意,最后一个将生成主要提交。 它与添加重大更改页脚具有相同的结果
按照Semantic Versioning 2.0.0 更新项目版本。
Release-It 将负责版本控制,您只需提供正确的类型即可提交:
- fix - to indicate a bug fix (PATCH) ex . v0.0.1
- feat - to indicate a new feature (MINOR) ex. v0.1.0
- chore - for updates that do not require a version bump (.gitignore, comments, etc.)
- docs - for updates to the documentation
- BREAKING CHANGE - regardless of type, indicates a Major release (MAJOR) ex. v1.0.0
访问 常规提交 获取更多示例。
发布 在上次提交/修改后运行以下命令。 然后用 Y/N 回答提示
yarn release
License
MIT
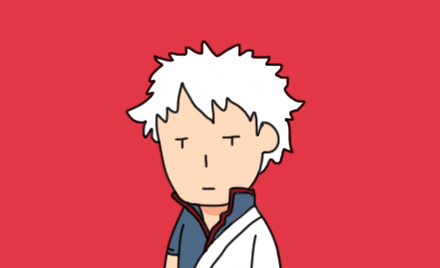
@2600hz/sds-react-native-components
Library that contains all sharable components for all react native applications development.
[](https://img.shields.io/npm/v/@2600hz/sds-react-native-components?style=flat-square) [](https://github.com/prettier/prettier)
2600Hz - Commio
SDS React Native Components Library
Installation
yarn add @2600hz/sds-react-native-components
Needed packages
If you don't already have them on your app, yarn will install the following packages needed for usability. These packages are added as peerDependencies
- @2600hz/commio-native-utilities - Contains useful js functions and shareable configurations
- @2600hz/sds-react-native-theme - Contains theme available for react native apps that use styled-components
- Styled-Components - Library to style react components
- react-native-svg - Provides SVG support to React Native on iOS and Android, and a compatibility layer for the web
- tslib - Contains all of the TypeScript helper functions. Even if your project doesn't use Typescript, you need this package for native-components to function properly
Packages considerations
If you already have those libraries on your app, then you need to make the following changes on configuration files for the package to function properly Do this on your App root folder
// metro.config.js
const modules = [
'@2600hz/commio-native-utilities',
'@2600hz/sds-react-native-theme',
'react',
'react-dom',
'react-native',
'react-native-svg',
'styled-components',
'tslib',
];
module.exports = (async () => {
return {
// ...
resolver: {
extraNodeModules: modules.reduce((acc, name) => {
acc[name] = path.join(__dirname, 'node_modules', name);
return acc;
}, {}),
},
// ...
};
})();
Now use that in webpack
file
// webpack.config.js
const metroConfig = require('./metro.config');
const node_modules = path.join(__dirname, 'node_modules');
module.exports = async function (env, argv) {
const config = await createExpoWebpackConfigAsync(env, argv);
const { resolver } = await metroConfig;
// We need to make sure that only one version is loaded for peerDependencies
// So we alias them to the versions in example's node_modules
Object.assign(config.resolve.alias, {
...resolver.extraNodeModules,
});
return config;
};
Styled-Components considerations We use a theme built for styled-components. Styled components can be used from two sources
- The one installed automatically when installing @2600hz/sds-react-native-components
- The one installed manually by you on your app
On the first case, components package will use the theme you set up on ThemeProvider, for your own components and the built-in components in the package. If you install styled-components on your app, components package won't use the theme you setup automatically. therefore you need to ensure only one installing of styled-components is used, the app one.
We fix this by doing the changes in metro-config.js and webpack-config.js showed previously.
React Native Svg considerations There should be only one version of react-native-svg installed, if you manually install it in your app, make sure you add it in the list of extraNodeModules
so we make sure only one version is used. Otherwise it will throw an error on execution.
Usage
It relies on styled-components, so it needs a theme set for the application. Get the theme from @2600hz/sds-react-native-theme You can set the DefaultTheme as the one from sds-react-native-theme
. Create a declaration file, name it for convention styled.d.ts
import {} from 'styled-components';
import { DefaultThemeProps } from '@2600hz/sds-react-native-theme';
declare module 'styled-components' {
export interface DefaultTheme extends DefaultThemeProps {}
}
Now use it in your entry point component
import { lightTheme } from '@2600hz/sds-react-native-theme';
<ThemeProvider theme={lightTheme}>
{/* <App ... /> */}
{children}
</ThemeProvider>
This theme will apply to your components and the ones coming from sds-react-native-components
Now in your components
import { Button, Telicon } from "@2600hz/sds-react-native-components";
// ...
<Button onPress={handleCall} title="Call" color="#aaffcc" />
<View>
<Telicon
name="volume-x"
size="xsmall"
fill="green"
fillSecondary="pink"
/>
</View>
Svg Files
The package already have components to treat svg xml/files. These are
- SvgFromXml - Renders an icon, it accepts a svg xml string or file (Internally calls the following two)
- SvgFromXmlString - Renders an icon, it accepts a svg xml string
- SvgFromXmlFile - Renders an icon, it accepts a svg xml file
Examples
const xml = `<svg viewBox="0 0 512 512"> <path d="M472.916..." /></svg>`;
<SvgFromXml xml={xml} size="small" fill="green" />
<SvgFromXmlString xml={xml} size="medium" fill="blue" />
// ...
import UserIcon from './app/assets/user.svg';
<SvgFromXml xml={UserIcon} size="xsmall" fill="yellow" />
<SvgFromXmlFile Xml={UserIcon} size="xlarge" fill="magenta" />
If you want to handle svg files on your own or need a different icon not included in telicon, then create a declarations.d.ts
(If you already have one, then name it differently) Add this content to file
declare module '*.svg' {
import { SvgXmlFileType } from '@2600hz/sds-react-native-components';
const content: SvgXmlFileType;
export default content;
}
Then you can import svg files this way
import UserIcon from './app/assets/user.svg';
<UserIcon width={100} height={100} fill="red" />
Use svgrrc configuration provided by @2600hz/commio-native-utilities to handle how svgs are converted
const { svgrrcBaseConfig } = require('@2600hz/commio-native-utilities');
module.exports = {
...svgrrcBaseConfig,
native: true,
};
We use svgo to configure how svg xml should be transformed to JSX. Look for the available plugins, so you can decide what attributes should be moved. For instance, we want all colors to be converted to strings if possible (Ex. #f00 to red)
const { svgrrcBaseConfig } = require('@2600hz/commio-native-utilities');
module.exports = {
...svgrrcBaseConfig,
native: true,
svgoConfig: {
plugins: [
{ convertColors: true },
...
]
}
};
Development
This project requires Node.js v14+ to run.
Using .nvmrc file helps to normalize node version used by all maintainers. If you are required to use version specified in this file, run these commands.
nvm use
nvm install
Use the package manager yarn v1+ to install dependencies and devDependencies.
yarn install
yarn
Create symlink Run the following command on package root folder, so you can consume on an app locally
yarn link
Watch project Build package whenever there is a change
yarn watch
Consume the package Both projects must be placed on same folder, otherwise won't work
yarn link @2600hz/sds-react-native-components
Considerations with packages installed
- Add package as alias in
babel.config.js
// babel.config.js
const path = require('path');
module.exports = function (api) {
api.cache(true);
return {
// ...
plugins: [
// ...
[
'module-resolver',
{
alias: {
// ...
// For development, we want to alias the library to the source
// Remember we have the package folder right next to the app
'@2600hz/sds-react-native-components': path.join(__dirname, '..', 'sds-react-native-components', 'src/index'),
},
},
],
],
};
};
- Blacklist peerDependencies to ensure only one version of packages is used (The one from App)
// metro.config.js
const path = require('path');
const blacklist = require('metro-config/src/defaults/blacklist');
const escape = require('escape-string-regexp');
// Assuming your package folder is named "sds-react-native-components" and it's located right next to the app folder
const root = path.resolve(__dirname, '..', 'sds-react-native-components');
const modules = [
'@2600hz/commio-native-utilities',
'@2600hz/sds-react-native-theme',
'react',
'react-dom',
'react-native',
'react-native-svg',
'styled-components',
'tslib',
];
module.exports = (async () => {
return {
projectRoot: __dirname,
watchFolders: [root],
resolver: {
// We need to make sure that only one version is loaded for peerDependencies
// So we blacklist them at the root, and alias them to the versions in example's node_modules
blacklistRE: blacklist(
modules.map(
(m) =>
new RegExp(`^${escape(path.join(root, 'node_modules', m))}\\/.*$`)
)
),
extraNodeModules: modules.reduce((acc, name) => {
acc[name] = path.join(__dirname, 'node_modules', name);
return acc;
}, {}),
},
};
})();
@2600hz/sds-telicon This package contains the xml definition of telicon svg, we provide SvgTelicon
and Telicon
components for apps to easily render icons on screen. Everytime sds-telicon gets updated, we need to bump its version on our components package, automatically will update Telicon components with new telicon.svg changes. If you want to manually convert telicon.svg to component run
yarn svg:jsx
Note: This command is executed every time "yarn install" runs
Build the package Automatically will run bob build
yarn build
Utilities
Format code using Eslint
yarn run lint:fix
Format code using Prettier
yarn run format
Format code using Prettier and Eslint
yarn run prettify
Check Typescript on files
yarn run typescript
Run full check
yarn run full-check
Tech
Components library uses a number of open source projects to work properly:
- React.js - JavaScript library for building user interfaces.
- React Native - JavaScript library for creating native apps for Android and IOS using React.
- Typescript - Strongly typed programming language which builds on JavaScript
- NodeJs - Allows execute javascript scripts on the terminal
- Storybook - Development environment for UI components
- Luxon - library for dealing with dates and times in JavaScript (better alternative than Moment.js)
- Styled-Components - Library to style react components
- react-native-svg - Provides SVG support to React Native on iOS and Android, and a compatibility layer for the web
- create-react-native-library - CLI to scaffold React Native libraries
- @2600hz/sds-react-native-theme - React Native Theme for Styled-components
- @2600hz/commio-native-utilities - Library written in js for shareable config files and common functions
Contributing
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
See the contributing guide to learn how to contribute to the repository and the development workflow.
Commits Commits must follow conventional commit format Make sure your messages look like the following examples
feat: Adding new badge component
fix: Touchable component not being exported correctly
fix!: Drop support for Typescript
Note that the last one will generate a Major commit. It has the same result as adding a breaking change footer
Follow Semantic Versioning 2.0.0 to update project version.
Release-It will take care of versioning, you just have to give the correct type to commit:
- fix - to indicate a bug fix (PATCH) ex . v0.0.1
- feat - to indicate a new feature (MINOR) ex. v0.1.0
- chore - for updates that do not require a version bump (.gitignore, comments, etc.)
- docs - for updates to the documentation
- BREAKING CHANGE - regardless of type, indicates a Major release (MAJOR) ex. v1.0.0
Visit Conventional Commits for more examples.
Make a release Run following command after your last commit/amend. Then answer with Y/N the prompt
yarn release
License
MIT