在 C++ 中使用 De Casteljau 算法绘制贝塞尔曲线,OpenGL
我正在尝试找到使用 decasteljau 算法生成贝塞尔曲线的方法,以完成我的一项作业。我能够使用正常方法生成贝塞尔曲线,但无法开始使用上述算法生成。如果有人可以建议我正确的方向或分享您拥有的任何代码,这将会有很大的帮助。我不只是按原样询问。我为此做了很多工作,并自己编写了以下代码来生成曲线。 我找到了一个网络小程序,它正是我所需要的。 (http://www2.mat.dtu.dk/people/J.Gravesen/cagd/decast.html)。建议我如何实现这一目标
#include <iostream>
using std::cerr;
using std::endl;
#include <stdlib.h>
//using std::exit;
#include <GL/glut.h> // GLUT stuff, includes OpenGL headers as well
#include <windows.h>
#include <math.h>
#include <gl/Gl.h>
#include <gl/Glu.h>
int SCREEN_HEIGHT = 480;
// Keep track of times clicked, on 3 clicks draw.
int NUMPOINTS = 0;
// Point class to keep it a little cleaner.
class Point {
public:
float x, y, z;
void setxy(float x2, float y2) { x = x2; y = y2; }
const Point & operator=(const Point &rPoint) {
x = rPoint.x;
y = rPoint.y;
z = rPoint.z;
return *this;
}
};
Point abc[4];
void myInit() {
glClearColor(0.0,0.0,0.0,0.0);
glColor3f(1.0,0.0,0.0);
glPointSize(4.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0.0,640.0,0.0,480.0);
}
void drawDot(int x, int y) {
glBegin(GL_POINTS);
glVertex2i(x,y);
glEnd();
glFlush();
}
void drawLine(Point p1, Point p2) {
glBegin(GL_LINES);
glVertex3f(p1.x, p1.y, p1.z);
glVertex3f(p2.x, p2.y, p2.z);
glEnd();
glFlush();
}
// Calculate the next bezier point.
Point drawBezier(Point A, Point B, Point C, Point D, double t) {
Point P;
P.x = pow((1 - t), 3) * A.x + 3 * t * pow((1 -t), 2) * B.x + 3 * (1-t) * pow(t, 2)* C.x + pow (t, 3)* D.x;
P.y = pow((1 - t), 3) * A.y + 3 * t * pow((1 -t), 2) * B.y + 3 * (1-t) * pow(t, 2)* C.y + pow (t, 3)* D.y;
P.z = pow((1 - t), 3) * A.z + 3 * t * pow((1 -t), 2) * B.z + 3 * (1-t) * pow(t, 2)* C.z + pow (t, 3)* D.z;
return P;
}
void myMouse(int button, int state, int x, int y) {
// If left button was clicked
if(button == GLUT_LEFT_BUTTON && state == GLUT_DOWN) {
// Store where the user clicked, note Y is backwards.
abc[NUMPOINTS].setxy((float)x,(float)(SCREEN_HEIGHT - y));
NUMPOINTS++;
// Draw the red dot.
drawDot(x, SCREEN_HEIGHT - y);
// If 3 points are drawn do the curve.
if(NUMPOINTS == 4) {
glColor3f(1.0,1.0,1.0);
// Draw two legs of the triangle
drawLine(abc[0], abc[1]);
drawLine(abc[1], abc[2]);
drawLine(abc[2], abc[3]);
//drawLine(abc[3], abc[4]);
Point POld = abc[0];
/* Draw each segment of the curve. Make t increment in
smaller amounts for a more detailed curve. */
for(double t = 0.0;t <= 1.0; t += 0.1) {
Point P = drawBezier(abc[0], abc[1], abc[2], abc[3], t);
drawLine(POld, P);
POld = P;
}
glColor3f(1.0,0.0,0.0);
NUMPOINTS = 0;
}
}
}
void myDisplay() {
glClear(GL_COLOR_BUFFER_BIT);
glFlush();
}
int main(int argc, char *argv[]) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);
glutInitWindowSize(640,480);
glutInitWindowPosition(100,150);
glutCreateWindow("Bezier Curve");
glutMouseFunc(myMouse);
glutDisplayFunc(myDisplay);
myInit();
glutMainLoop();
return 0;
}
I am trying to find the way to generate bezier curve using de casteljau algorithm for one of my assignment here. I am able to generate bezier curve using normal method but unable to start on generating using the above algorithm. It will be of great help if someone can suggest me to right direction or share any piece of code you have. I am not just asking as is. I worked a lot on it and wrote the following code myself to generate the curve.
I found a web applet which does exactly i needed. (http://www2.mat.dtu.dk/people/J.Gravesen/cagd/decast.html). suggest me how to achieve that
#include <iostream>
using std::cerr;
using std::endl;
#include <stdlib.h>
//using std::exit;
#include <GL/glut.h> // GLUT stuff, includes OpenGL headers as well
#include <windows.h>
#include <math.h>
#include <gl/Gl.h>
#include <gl/Glu.h>
int SCREEN_HEIGHT = 480;
// Keep track of times clicked, on 3 clicks draw.
int NUMPOINTS = 0;
// Point class to keep it a little cleaner.
class Point {
public:
float x, y, z;
void setxy(float x2, float y2) { x = x2; y = y2; }
const Point & operator=(const Point &rPoint) {
x = rPoint.x;
y = rPoint.y;
z = rPoint.z;
return *this;
}
};
Point abc[4];
void myInit() {
glClearColor(0.0,0.0,0.0,0.0);
glColor3f(1.0,0.0,0.0);
glPointSize(4.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0.0,640.0,0.0,480.0);
}
void drawDot(int x, int y) {
glBegin(GL_POINTS);
glVertex2i(x,y);
glEnd();
glFlush();
}
void drawLine(Point p1, Point p2) {
glBegin(GL_LINES);
glVertex3f(p1.x, p1.y, p1.z);
glVertex3f(p2.x, p2.y, p2.z);
glEnd();
glFlush();
}
// Calculate the next bezier point.
Point drawBezier(Point A, Point B, Point C, Point D, double t) {
Point P;
P.x = pow((1 - t), 3) * A.x + 3 * t * pow((1 -t), 2) * B.x + 3 * (1-t) * pow(t, 2)* C.x + pow (t, 3)* D.x;
P.y = pow((1 - t), 3) * A.y + 3 * t * pow((1 -t), 2) * B.y + 3 * (1-t) * pow(t, 2)* C.y + pow (t, 3)* D.y;
P.z = pow((1 - t), 3) * A.z + 3 * t * pow((1 -t), 2) * B.z + 3 * (1-t) * pow(t, 2)* C.z + pow (t, 3)* D.z;
return P;
}
void myMouse(int button, int state, int x, int y) {
// If left button was clicked
if(button == GLUT_LEFT_BUTTON && state == GLUT_DOWN) {
// Store where the user clicked, note Y is backwards.
abc[NUMPOINTS].setxy((float)x,(float)(SCREEN_HEIGHT - y));
NUMPOINTS++;
// Draw the red dot.
drawDot(x, SCREEN_HEIGHT - y);
// If 3 points are drawn do the curve.
if(NUMPOINTS == 4) {
glColor3f(1.0,1.0,1.0);
// Draw two legs of the triangle
drawLine(abc[0], abc[1]);
drawLine(abc[1], abc[2]);
drawLine(abc[2], abc[3]);
//drawLine(abc[3], abc[4]);
Point POld = abc[0];
/* Draw each segment of the curve. Make t increment in
smaller amounts for a more detailed curve. */
for(double t = 0.0;t <= 1.0; t += 0.1) {
Point P = drawBezier(abc[0], abc[1], abc[2], abc[3], t);
drawLine(POld, P);
POld = P;
}
glColor3f(1.0,0.0,0.0);
NUMPOINTS = 0;
}
}
}
void myDisplay() {
glClear(GL_COLOR_BUFFER_BIT);
glFlush();
}
int main(int argc, char *argv[]) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);
glutInitWindowSize(640,480);
glutInitWindowPosition(100,150);
glutCreateWindow("Bezier Curve");
glutMouseFunc(myMouse);
glutDisplayFunc(myDisplay);
myInit();
glutMainLoop();
return 0;
}
如果你对这篇内容有疑问,欢迎到本站社区发帖提问 参与讨论,获取更多帮助,或者扫码二维码加入 Web 技术交流群。

绑定邮箱获取回复消息
由于您还没有绑定你的真实邮箱,如果其他用户或者作者回复了您的评论,将不能在第一时间通知您!
发布评论
评论(2)
对我有用,有什么问题吗?
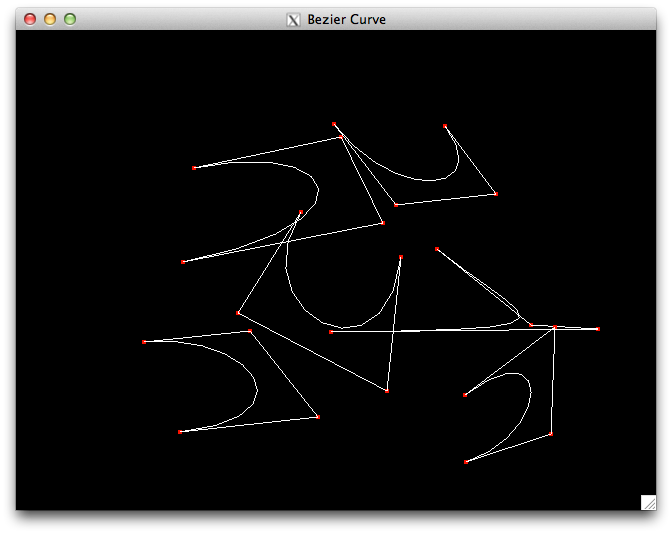
我不会将此作为答案发布,但我无法在评论中发布图像
works for me, what is the problem?
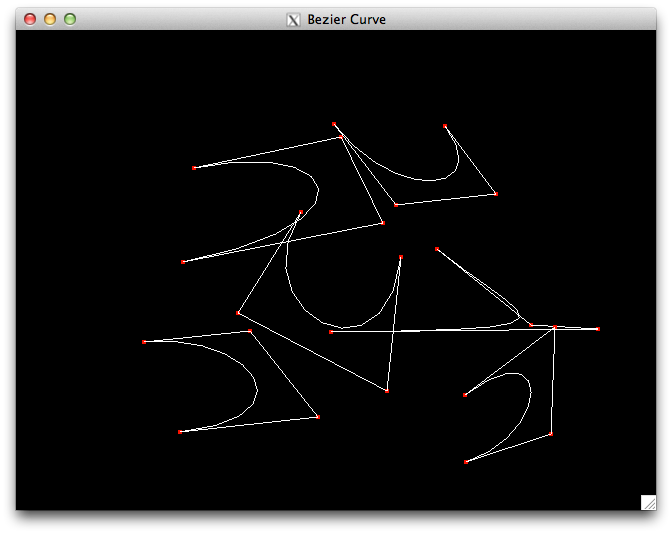
I wouldn't post this as an answer, but I can't post an image in a comment
通过此链接,您可以找到交互式 JavaScript 实现。
只需注意点
AB
是如何使用(( 1-t)*A + t*B)
并查看其他点 (BC
、CD
、ABC
、BCD
最后是ABCD
)类似地计算。您可以将
A
、B
、C
和D
以及点AB
拖动到查看构造如何根据参数 t 进行工作。Following this link you can find an interactive javascript implementation.
Just note how point
AB
is for example computed using((1-t)*A + t*B)
and see how other points (BC
,CD
,ABC
,BCD
and finallyABCD
) are similarly computed.You can drag
A
,B
,C
andD
and the pointAB
to see how the construction works depending on the parametert
.