如何向绘图添加悬停注释
我正在使用 matplotlib 制作散点图。散点图上的每个点都与一个命名对象相关联。当我将光标悬停在与该对象关联的散点图上的点上时,我希望能够看到该对象的名称。特别是,如果能够快速查看异常值点的名称,那就太好了。我在此处搜索时找到的最接近的东西是注释命令,但这似乎在绘图上创建了一个固定标签。不幸的是,根据我拥有的点数,如果我标记每个点,散点图将无法读取。有谁知道如何创建仅当光标悬停在该点附近时才出现的标签?
I am using matplotlib to make scatter plots. Each point on the scatter plot is associated with a named object. I would like to be able to see the name of an object when I hover my cursor over the point on the scatter plot associated with that object. In particular, it would be nice to be able to quickly see the names of the points that are outliers. The closest thing I have been able to find while searching here is the annotate command, but that appears to create a fixed label on the plot. Unfortunately, with the number of points that I have, the scatter plot would be unreadable if I labeled each point. Does anyone know of a way to create labels that only appear when the cursor hovers in the vicinity of that point?
如果你对这篇内容有疑问,欢迎到本站社区发帖提问 参与讨论,获取更多帮助,或者扫码二维码加入 Web 技术交流群。

绑定邮箱获取回复消息
由于您还没有绑定你的真实邮箱,如果其他用户或者作者回复了您的评论,将不能在第一时间通知您!
发布评论
评论(13)
下面的代码使用散点,并在悬停在散点上时显示注释。
因为人们还希望将此解决方案用于线图而不是散点图,所以以下将是用于图的相同解决方案(适用)略有不同)。
如果有人正在寻找双轴线条的解决方案,请参阅 如何在将鼠标悬停在多轴中的某个点上时显示标签?
以防有人正在寻找 bar 的解决方案情节,请参考例如这个答案。
Here is a code that uses a scatter and shows an annotation upon hovering over the scatter points.
Because people also want to use this solution for a line
plot
instead of a scatter, the following would be the same solution forplot
(which works slightly differently).In case someone is looking for a solution for lines in twin axes, refer to How to make labels appear when hovering over a point in multiple axis?
In case someone is looking for a solution for bar plots, please refer to e.g. this answer.
此解决方案在悬停一行时有效,无需单击它:
This solution works when hovering a line without the need to click it:
pip
的说明。%matplotlib qt
的操作将打开交互式绘图。请参阅如何在 IPython 笔记本中打开交互式 matplotlib 窗口?python 3.10 中测试
、pandas 1.4.2、matplotlib 3.5.1、seaborn 0.11.2熊猫
Seaborn
sns.lineplot
,以及图形级绘图,例如sns.relplot
。mplcursors
package is probably the easiest option.pip
.%matplotlib qt
in a cell will turn on interactive plotting. See How can I open the interactive matplotlib window in IPython notebook?python 3.10
,pandas 1.4.2
,matplotlib 3.5.1
,seaborn 0.11.2
Pandas
Seaborn
sns.lineplot
, and figure-level plots likesns.relplot
.来自 http://matplotlib.sourceforge.net/examples/event_handling/pick_event_demo.html :
matplotlib 中的点和线工具提示?
From http://matplotlib.sourceforge.net/examples/event_handling/pick_event_demo.html :
Point and line tooltips in matplotlib?
其他答案没有满足我在 Jupyter 内联 matplotlib 图的最新版本中正确显示工具提示的需求。但这是有效的:
当用鼠标移动到某个点时,会导致如下图所示的结果:
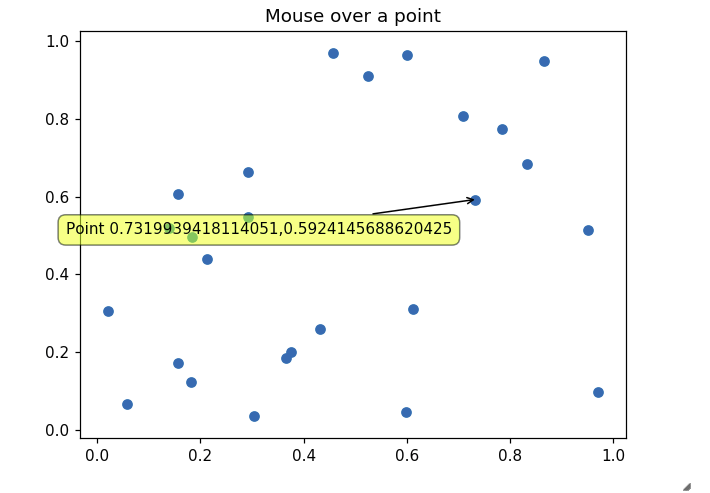
The other answers did not address my need for properly showing tooltips in a recent version of Jupyter inline matplotlib figure. This one works though:
Leading to something like the following picture when going over a point with mouse:
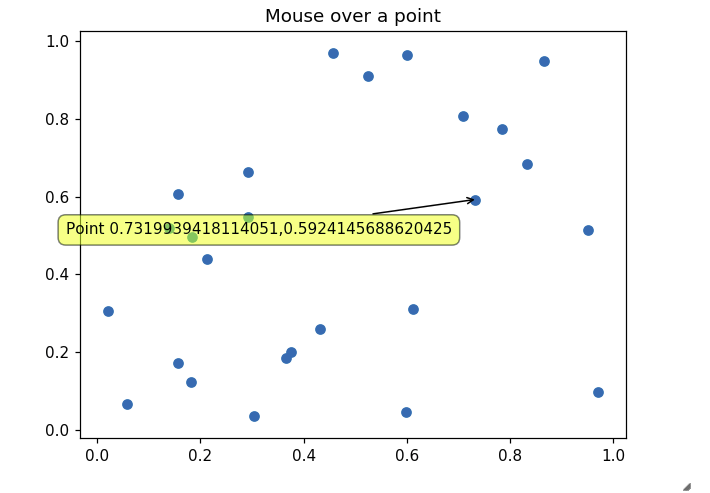
对 http://matplotlib.org/users/shell.html:
这绘制了一条直线图,正如 Sohaib 所要求的那样
A slight edit on an example provided in http://matplotlib.org/users/shell.html:
This plots a straight line plot, as Sohaib was asking
mplcursors 为我工作。 mplcursors 为 matplotlib 提供可点击的注释。它深受 mpldatacursor (https://github.com/joferkington/mpldatacursor) 的启发,有很多简化的API
mplcursors worked for me. mplcursors provides clickable annotation for matplotlib. It is heavily inspired from mpldatacursor (https://github.com/joferkington/mpldatacursor), with a much simplified API
mpld3 为我解决了这个问题。
您可以查看以下示例: https://mpld3.github.io/examples/scatter_tooltip.html< /a>
mpld3 solves it for me.
You can check this example: https://mpld3.github.io/examples/scatter_tooltip.html
我制作了一个多行注释系统添加到: https://stackoverflow.com/a/47166787/10302020 。
对于最新版本:
https://github.com/AidenBurgess/MultiAnnotationLineGraph
只需更改底部的数据即可。
I have made a multi-line annotation system to add to: https://stackoverflow.com/a/47166787/10302020.
for the most up to date version:
https://github.com/AidenBurgess/MultiAnnotationLineGraph
Simply change the data in the bottom section.
在 matplotlib 状态栏中显示对象信息
特点
scatter
、plot
,add_patch
)代码
showing object information in matplotlib statusbar
Features
scatter
,plot
,add_patch
)Code
基于 Markus Dutschke”和“ImportanceOfBeingErnest”,我(imo)简化了代码并使其更加模块化。
而且这不需要安装额外的软件包。
给出以下结果:
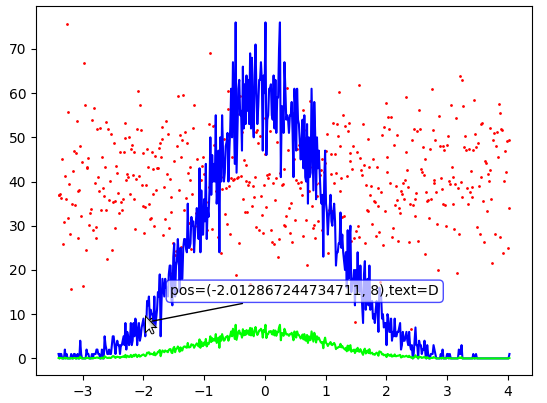
Based off Markus Dutschke" and "ImportanceOfBeingErnest", I (imo) simplified the code and made it more modular.
Also this doesn't require additional packages to be installed.
Giving the following result:
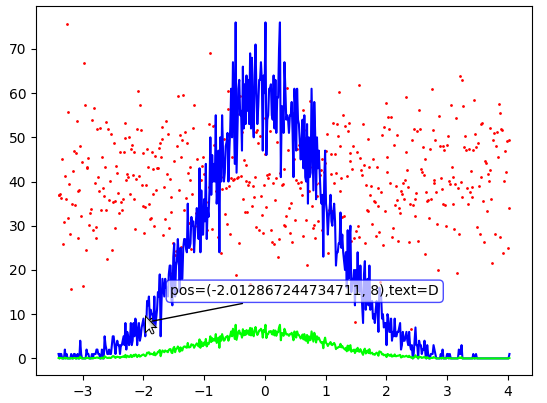
我已经调整了 ImportanceOfBeingErnest 的答案来使用补丁和类。特点:
注意:对于我的应用程序,重叠是不相关,因此一次仅显示一个对象的名称。如果您愿意,可以随意扩展到多个对象,这并不太难。
使用
实现
I have adapted ImportanceOfBeingErnest's answer to work with patches and classes. Features:
Note: For my applications, the overlap is not relevant, thus only one object's name is displayed at a time. Feel free to extend to multiple objects if you wish, it is not too hard.
Usage
Implementation
另一种选择是使用 Plotly,它非常直观且易于使用,并且具有能够将具有悬停行为的绘图保存为
html
文件的巨大优势。下面是一些直观的例子:
https://plotly.com/python/line-and-scatter/
并且这是详细的文档: https://plotly.com/python-api-reference/ generated/plotly.express.scatter.html
Yet another alternative is to use Plotly which is really intuitive and easy to use and has the great advantage of being able to save your plot with the hovering behavior as an
html
file.Here are some intuitive examples:
https://plotly.com/python/line-and-scatter/
And here is the detailed documentation: https://plotly.com/python-api-reference/generated/plotly.express.scatter.html