如何以编程方式将 UIToolbar 添加到 iOS 应用程序?
似乎找不到按照问题标题描述的教程。我想了解 UIToolbar 需要在哪里声明以及如何将其放到我的视图层上。
如果你对这篇内容有疑问,欢迎到本站社区发帖提问 参与讨论,获取更多帮助,或者扫码二维码加入 Web 技术交流群。

绑定邮箱获取回复消息
由于您还没有绑定你的真实邮箱,如果其他用户或者作者回复了您的评论,将不能在第一时间通知您!
似乎找不到按照问题标题描述的教程。我想了解 UIToolbar 需要在哪里声明以及如何将其放到我的视图层上。
由于您还没有绑定你的真实邮箱,如果其他用户或者作者回复了您的评论,将不能在第一时间通知您!
接受
或继续使用网站,即表示您同意使用 Cookies 和您的相关数据。
发布评论
评论(7)
如果您使用的是
UINavigationController
,那么默认情况下工具栏会附带它。您可以使用以下代码行添加它:
要将按钮添加到工具栏,您可以使用以下代码:
flexibleItem
用于保持我们上面创建的两个按钮之间的适当距离。现在您可以添加这三个项目以使它们在您的视图中可见。
我希望它对你有用。
If you're using
UINavigationController
then the toolbar comes with it by default.You can add it using following line of code:
And to add button to your Toolbar you can use following code:
flexibleItem
is used to maintain proper distance between the two buttons that we have created above.Now you can add these three items in order to make them visible on your view.
I hope it works for you.
UIToolbar
是UIView
的子类,因此您的问题的简短答案是:就像任何其他视图一样。具体来说,这是如何以编程方式创建工具栏的示例。此代码片段中的上下文是视图控制器的
viewDidLoad
。请参阅 UIToolbar 和 UIBarButtonItem< /a> 文档以获取详细信息。
UIToolbar
is a subclass ofUIView
, so the short answer to your question is: just like any other view.Specifically, this is an example of how to programmatically create a toolbar. The context in this snippet is
viewDidLoad
of a view controller.See UIToolbar and UIBarButtonItem documentation for details.
iOS 11+ SWIFT 4 + Xcode 9 + 约束
适用于横向 + 纵向
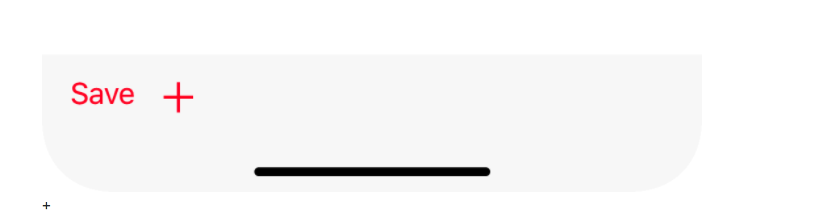
iOS 11+ SWIFT 4 + Xcode 9 + Constraints
Works for both landscape + Portrait
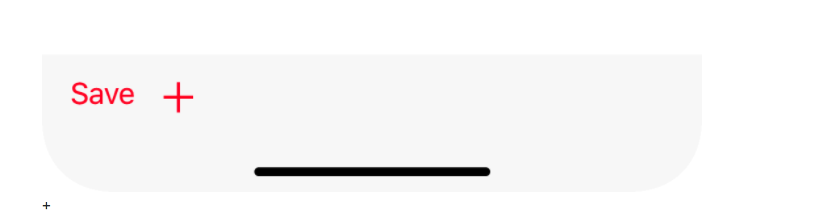
要在底部显示工具栏,左侧的两个按钮和右侧的另一个按钮之间有空间
注意:要在按钮之间留出空间,我们添加如下行
< strong>并添加间隔符
To show the Toolbar at the bottom with space between two button on at Left Side , and another at Right side
Note: To Give space between to Button we add line as below
and add spacer to the
尝试这个简单的方法:
Try this simple Method:
这就是您在应用中实现
UIToolbar
的方式。您还应该必须实现以下委托方法:
This is how you implement a
UIToolbar
in your app.You should also have to implement the following delegate methods:
Swift 5:
结果:
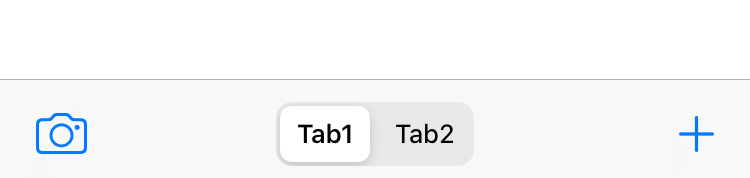
代码:
Swift 5:
Result:
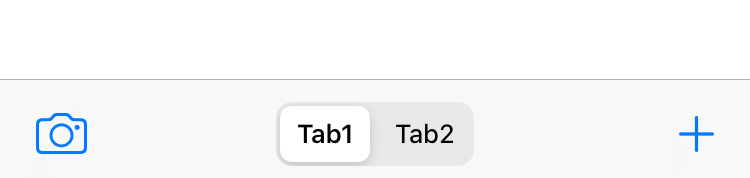
Code: