scala 模式匹配 - 变量
当我阅读这个(第14页)时,我遇到了这个算法:
function fib2(n)
if n = 0 return 0
create an array f[0 : : : n]
f[0] = 0, f[1] = 1
for i = 2 : : : n:
f[i] = f[i 1] + f[i 2]
return f[n]
如果我想在 Scala 中使用模式匹配来实现这一点,有没有办法在模式匹配部分创建一个列表,以便在最终的返回语句中使用它?
这些都是很好的答案,但我想我仍然想知道是否可以定义一个仅在模式匹配中使用的变量。我知道你可以在 Haskell 中做到这一点,但我想知道它在 Scala 中是否可行。
While I was reading this (page 14) I came across this algorithm:
function fib2(n)
if n = 0 return 0
create an array f[0 : : : n]
f[0] = 0, f[1] = 1
for i = 2 : : : n:
f[i] = f[i 1] + f[i 2]
return f[n]
If I wanted to implement this in Scala using pattern matching, is there a way to create a List in the pattern match part in order to use it in the final return statement?
these are great answers, but I think I'd still like to know if it's possible to define a variable that you only use in your pattern match. I know you can do it in Haskell, but I'm wondering if it's doable in Scala.
如果你对这篇内容有疑问,欢迎到本站社区发帖提问 参与讨论,获取更多帮助,或者扫码二维码加入 Web 技术交流群。

绑定邮箱获取回复消息
由于您还没有绑定你的真实邮箱,如果其他用户或者作者回复了您的评论,将不能在第一时间通知您!
发布评论
评论(4)
fib.take(n).last 将返回结果
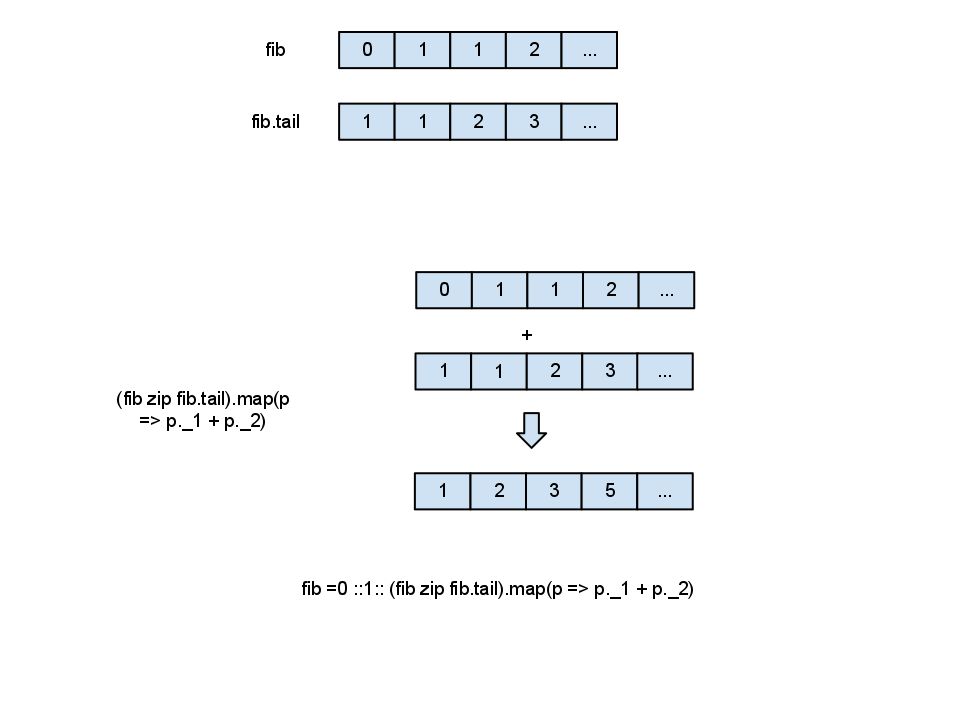
另一个基于流的解决方案。它定义了一个无限的斐波那契数列。是的,它是救援和无限定义,但所有计算都是在调用 take 时执行的。
只需在这里查看更多有趣的代码即可。
链接
fib.take(n).last will return the result
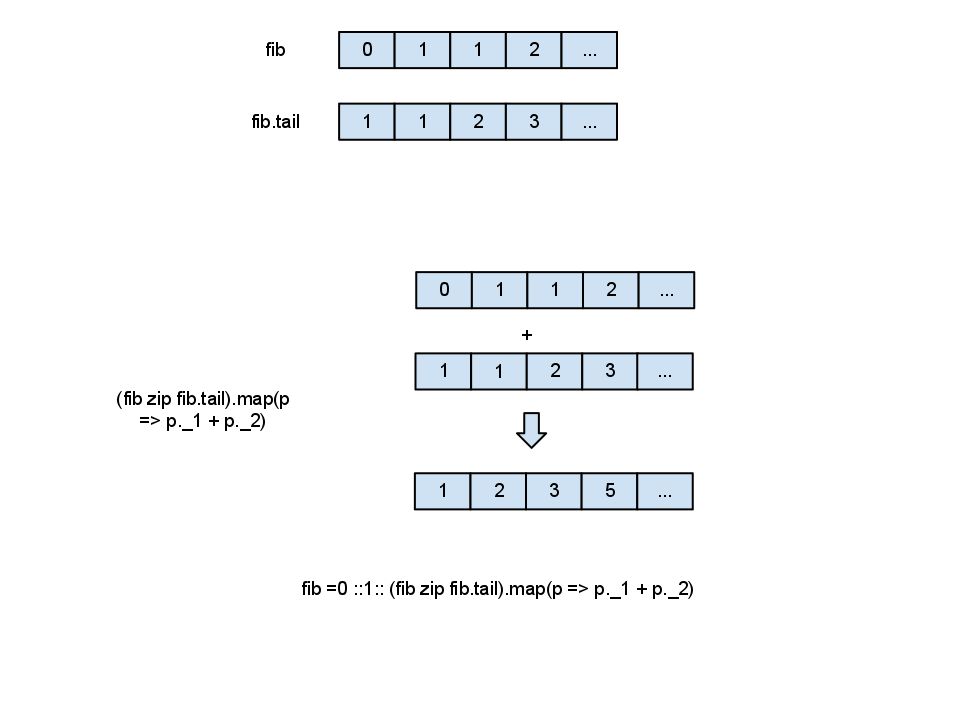
another stream based solution. It defines a infinite Fibonacci sequence. Yes it is rescue and infinite definition, but all computations are performed while take is called.
just check here for more interesting code.
link
我认为这里没有太多必要的模式匹配。 Scala 的直接转换看起来基本相同:创建一个数组
f
并循环索引2 直到 n
。如果您想要更高级,惰性流怎么样?
I don't see much need for pattern matching here. The straightforward translation to Scala would look basically the same: create an array
f
and loop over indices2 until n
.If you want to get fancier, how about a lazy stream?
这是 Jeela 解决方案的重构。我认为最好总是用头脑来工作,因为它要快得多。最后的
逆转
并没有造成太大伤害。Here is a refactoring of jeela's solution. I think it's better to work always with the head, as it is much faster. The final
reverse
doesn't hurt much.我认为使用惰性流是更好的方法,但只是为了展示我的肌肉:
I think using lazy streams is better approach but just to flex my muscles: