/**
* This will write a custom ID3 tag (TXXX).
* This works only with MP3 files (Flac with ID3-Tag not tested).
* @param description The description of the custom tag i.e. "catalognr"
* There can only be one custom TXXX tag with that description in one MP3 file
* @param text The actual text to be written into the new tag field
* @return True if the tag has been properly written, false otherwise
*/
public boolean setCustomTag(AudioFile audioFile, String description, String text){
FrameBodyTXXX txxxBody = new FrameBodyTXXX();
txxxBody.setDescription(description);
txxxBody.setText(text);
// Get the tag from the audio file
// If there is no ID3Tag create an ID3v2.3 tag
Tag tag = audioFile.getTagOrCreateAndSetDefault();
// If there is only a ID3v1 tag, copy data into new ID3v2.3 tag
if(!(tag instanceof ID3v23Tag || tag instanceof ID3v24Tag)){
Tag newTagV23 = null;
if(tag instanceof ID3v1Tag){
newTagV23 = new ID3v23Tag((ID3v1Tag)audioFile.getTag()); // Copy old tag data
}
if(tag instanceof ID3v22Tag){
newTagV23 = new ID3v23Tag((ID3v11Tag)audioFile.getTag()); // Copy old tag data
}
audioFile.setTag(newTagV23);
}
AbstractID3v2Frame frame = null;
if(tag instanceof ID3v23Tag){
frame = new ID3v23Frame("TXXX");
}
else if(tag instanceof ID3v24Tag){
frame = new ID3v24Frame("TXXX");
}
frame.setBody(txxxBody);
try {
tag.addField(frame);
} catch (FieldDataInvalidException e) {
e.printStackTrace();
return false;
}
try {
audioFile.commit();
} catch (CannotWriteException e) {
e.printStackTrace();
return false;
}
return true;
}
You should have a look at the library jAudioTagger. Below is an example that writes a custom tag (TXXX) to an audio file:
/**
* This will write a custom ID3 tag (TXXX).
* This works only with MP3 files (Flac with ID3-Tag not tested).
* @param description The description of the custom tag i.e. "catalognr"
* There can only be one custom TXXX tag with that description in one MP3 file
* @param text The actual text to be written into the new tag field
* @return True if the tag has been properly written, false otherwise
*/
public boolean setCustomTag(AudioFile audioFile, String description, String text){
FrameBodyTXXX txxxBody = new FrameBodyTXXX();
txxxBody.setDescription(description);
txxxBody.setText(text);
// Get the tag from the audio file
// If there is no ID3Tag create an ID3v2.3 tag
Tag tag = audioFile.getTagOrCreateAndSetDefault();
// If there is only a ID3v1 tag, copy data into new ID3v2.3 tag
if(!(tag instanceof ID3v23Tag || tag instanceof ID3v24Tag)){
Tag newTagV23 = null;
if(tag instanceof ID3v1Tag){
newTagV23 = new ID3v23Tag((ID3v1Tag)audioFile.getTag()); // Copy old tag data
}
if(tag instanceof ID3v22Tag){
newTagV23 = new ID3v23Tag((ID3v11Tag)audioFile.getTag()); // Copy old tag data
}
audioFile.setTag(newTagV23);
}
AbstractID3v2Frame frame = null;
if(tag instanceof ID3v23Tag){
frame = new ID3v23Frame("TXXX");
}
else if(tag instanceof ID3v24Tag){
frame = new ID3v24Frame("TXXX");
}
frame.setBody(txxxBody);
try {
tag.addField(frame);
} catch (FieldDataInvalidException e) {
e.printStackTrace();
return false;
}
try {
audioFile.commit();
} catch (CannotWriteException e) {
e.printStackTrace();
return false;
}
return true;
}
So all you have to do is write 128 bytes to the end of the file. Starting with TAG, followed by 30 bytes for the title, 30 for the artist, etc. The genre list can be found here.
If you want more tags (like album cover, lyrics, and much more) you need to use ID3v2. It works basically the same but is a little bit more complex, more info under https://id3.org/id3v2.3.0
发布评论
评论(3)
您应该查看一下库 jAudioTagger。下面是将自定义标签 (TXXX) 写入音频文件的示例:
You should have a look at the library jAudioTagger. Below is an example that writes a custom tag (TXXX) to an audio file:
这个库:
http://javamusictag.sourceforge.net/
提供了一种读取和写入 id3 标签的方法。
This library:
http://javamusictag.sourceforge.net/
provides a means of reading and writing id3 tags.
因为我不想使用库,所以我最终自己编写了一个标签编辑器。仅适用于 ID3v1 标签。
完整代码
这里是它的工作原理:
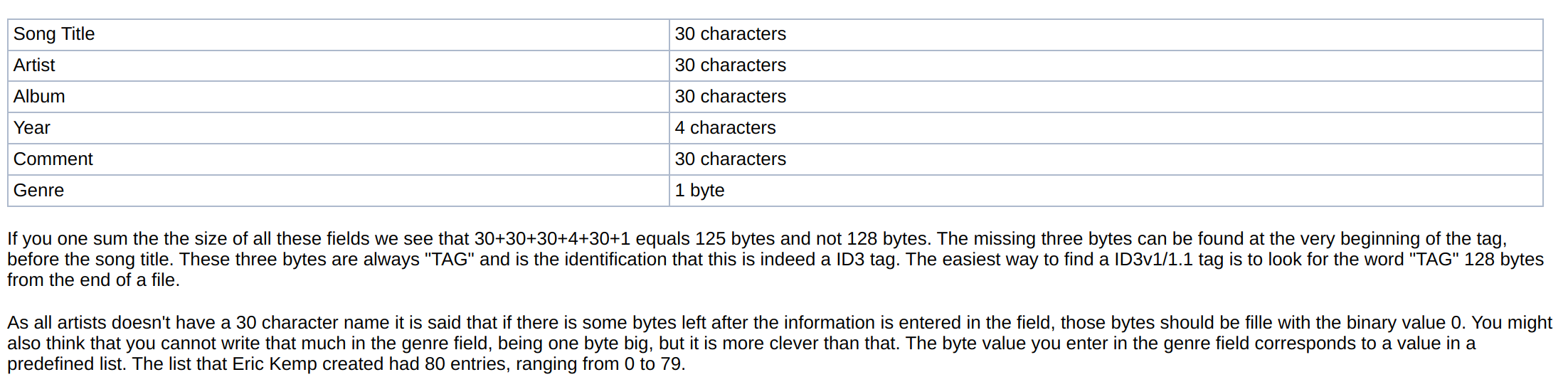
因此,您所要做的就是将 128 字节写入文件末尾。以
TAG
开始,后面是30个字节的标题,30个字节的艺术家等。流派列表可以在此处。如果您想要更多标签(例如专辑封面、歌词等等),您需要使用 ID3v2。它的工作原理基本相同,但稍微复杂一点,更多信息请参见 https://id3.org/id3v2。 3.0
I ended up coding a tag editor myself since I didn't want to use a library. It's for ID3v1 tags only.
Full Code
Here's how it works:
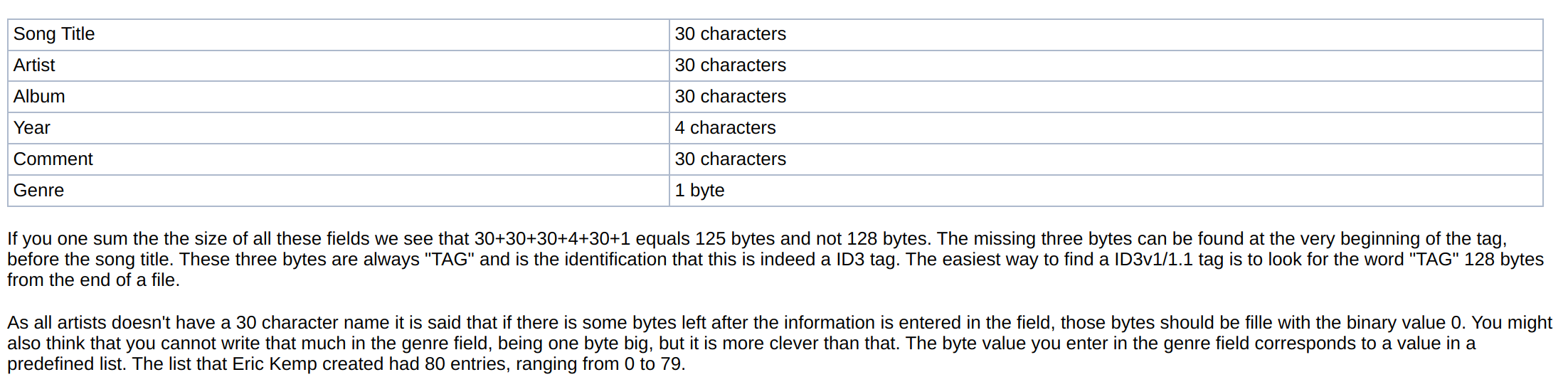
So all you have to do is write 128 bytes to the end of the file. Starting with
TAG
, followed by 30 bytes for the title, 30 for the artist, etc. The genre list can be found here.If you want more tags (like album cover, lyrics, and much more) you need to use ID3v2. It works basically the same but is a little bit more complex, more info under https://id3.org/id3v2.3.0