迭代器和可迭代器有什么区别以及如何使用它们?
我是 Java 新手,我真的对迭代器和可迭代感到困惑。谁能给我解释一下并举一些例子吗?
I am new in Java and I'm really confused with iterator and iterable. Can anyone explain to me and give some examples?
如果你对这篇内容有疑问,欢迎到本站社区发帖提问 参与讨论,获取更多帮助,或者扫码二维码加入 Web 技术交流群。

绑定邮箱获取回复消息
由于您还没有绑定你的真实邮箱,如果其他用户或者作者回复了您的评论,将不能在第一时间通知您!
发布评论
评论(15)
Iterable
是一系列可迭代元素的简单表示。它没有任何迭代状态,例如“当前元素”。相反,它有一种生成Iterator
的方法。Iterator
是具有迭代状态的对象。它允许您使用hasNext()
检查是否有更多元素,并使用next()
移动到下一个元素(如果有)。通常,
Iterable
应该能够生成任意数量的有效Iterator
。An
Iterable
is a simple representation of a series of elements that can be iterated over. It does not have any iteration state such as a "current element". Instead, it has one method that produces anIterator
.An
Iterator
is the object with iteration state. It lets you check if it has more elements usinghasNext()
and move to the next element (if any) usingnext()
.Typically, an
Iterable
should be able to produce any number of validIterator
s.Iterable
的实现是提供自身的Iterator
的实现:迭代器是一种简单的方法,允许某些人在没有分配权限的情况下循环访问数据集合(尽管具有能力)删除)。
请参阅 Javadoc。
An implementation of
Iterable
is one that provides anIterator
of itself:An iterator is a simple way of allowing some to loop through a collection of data without assignment privileges (though with ability to remove).
See Javadoc.
我将以 ArrayList 为例来回答这个问题,以帮助您更好地理解。Iterable
也就是说,你可以看到这样的关系
<块引用>
'Iterable <- Collection <- List <- ArrayList'
。
Iterable、Collection 和 List 只是声明了抽象方法“iterator()”,而 ArrayList 单独实现了它。
“iterator()”方法返回一个实现“Iterator”的类“Itr”的对象。
现在,清楚了吗? :)
I will answer the question especially about ArrayList as an example in order to help you understand better..
That is, you could see the relationship like
.
And Iterable, Collection and List just declare abstract method 'iterator()' and ArrayList alone implements it.
'iterator()' method returns an object of class 'Itr' which implements 'Iterator'.
Now, is it clear? :)
我知道这是一个老问题,但是对于任何读到这篇文章的人来说,如果他们遇到同样的问题,并且可能对所有术语感到不知所措,这里有一个很好的、简单的类比,可以帮助您理解迭代器和迭代器之间的区别
:公共图书馆。老派。与纸质书。是的,就是那种图书馆。
装满书的书架就像是一个可迭代对象。可以看到书架上排着长长的一排书。你可能不知道有多少本,但你可以看出,这是一本很长的藏书。
图书管理员就像迭代器。他可以随时指出一本特定的书。他可以在他指向的位置插入/删除/修改/阅读该书。每次你喊“下一本!”时,他都会按顺序一次指向每一本书。给他。所以,你通常会问他:“有下一个吗?”,他会说“是”,你说“下一个!”他会指着下一本书。他还知道什么时候到达了书架的末尾,因此当你问:“有下一个吗?”他会说“不”。
我知道这有点傻,但我希望这会有所帮助。
I know this is an old question, but for anybody reading this who is stuck with the same question and who may be overwhelmed with all the terminology, here's a good, simple analogy to help you understand this distinction between iterables and iterators:
Think of a public library. Old school. With paper books. Yes, that kind of library.
A shelf full of books would be like an iterable. You can see the long line of books in the shelf. You may not know how many, but you can see that it is a long collection of books.
The librarian would be like the iterator. He can point to a specific book at any moment in time. He can insert/remove/modify/read the book at that location where he's pointing. He points, in sequence, to each book at a time every time you yell out "next!" to him. So, you normally would ask him: "has Next?", and he'll say "yes", to which you say "next!" and he'll point to the next book. He also knows when he's reached the end of the shelf, so that when you ask: "has Next?" he'll say "no".
I know it's a bit silly, but I hope this helps.
如果集合是可迭代的,则可以使用迭代器对其进行迭代(因此可以在 foreach 循环中使用)。迭代器是将迭代集合的实际对象。
If a collection is iterable, then it can be iterated using an iterator (and consequently can be used in a for each loop.) The iterator is the actual object that will iterate through the collection.
实现 Iterable 接口允许对象成为“foreach”语句的目标。
迭代器是一个接口,它具有迭代元素的实现。 Iterable是一个提供Iterator的接口。
Implementing Iterable interface allows an object to be the target of the "foreach" statement.
Iterator is an interface, which has implementation for iterate over elements. Iterable is an interface which provides Iterator.
最重要的考虑因素是所讨论的项目是否应该能够被遍历多次。这是因为您始终可以通过再次调用 iterator() 来倒回 Iterable,但无法倒回 Iterator。
The most important consideration is whether the item in question should be able to be traversed more than once. This is because you can always rewind an Iterable by calling iterator() again, but there is no way to rewind an Iterator.
如上所述 这里,引入了“Iterable”以便能够在
foreach
循环中使用。实现了 Iterable 接口的类可以被迭代。Iterator 是管理 Iterable 迭代的类。它维护当前迭代中所处的状态,并知道下一个元素是什么以及如何获取它。
As explained here, The “Iterable” was introduced to be able to use in the
foreach
loop. A class implementing the Iterable interface can be iterated over.Iterator is class that manages iteration over an Iterable. It maintains a state of where we are in the current iteration, and knows what the next element is and how to get it.
考虑一个有 10 个苹果的例子。
当它实现Iterable时,就像将每个苹果放入从1到10的盒子中,并返回一个可用于导航的迭代器。
通过实现迭代器,我们可以获取任何苹果,下一个框中的苹果等。
因此,实现 iterable 可以提供一个迭代器来导航其元素,尽管要导航,需要实现迭代器。
Consider an example having 10 apples.
When it implements Iterable, it is like putting each apple in boxes from 1 to 10 and return an iterator which can be used to navigate.
By implementing iterator, we can get any apple, apple in next boxes etc.
So implementing iterable gives an iterator to navigate its elements although to navigate, iterator needs to be implemented.
问题:Iterable 和 Iterator 之间的区别?
Ans:
iterable:与forEach循环有关
iterator:是与 Collection 相关的
forEach 循环的目标元素应该是可迭代的。
Collection Iterable中一一获取对象
我们可以使用Iterator从java.ḷang包中的
java.util 包中存在的迭代器
仅包含一种方法 iterator()
包含三个方法 hasNext()、next()、remove()
1.5版本引入
1.2版本引入
Question:Difference between Iterable and Iterator?
Ans:
iterable: It is related to forEach loop
iterator: Is is related to Collection
The target element of the forEach loop shouble be iterable.
We can use Iterator to get the object one by one from the Collection
Iterable present in java.ḷang package
Iterator present in java.util package
Contains only one method iterator()
Contains three method hasNext(), next(), remove()
Introduced in 1.5 version
Introduced in 1.2 version
基本上来说,两者的关系非常密切。
将Iterator视为一个接口,它可以帮助我们借助一些未定义的方法(例如hasNext()、next()和remove())遍历集合
。另一方面,Iterable 是另一个接口,如果由类实现,则强制该类成为 Iterable,并且是 For-Each 构造的目标。
它只有一个名为 iterator() 的方法,该方法来自 Iterator 接口本身。
当集合是可迭代的时,就可以使用迭代器对其进行迭代。
如需了解,请访问这些:
可迭代: http://grepcode.com/file/repository.grepcode.com/java/root/jdk/openjdk/6-b14/java/lang/Iterable.java
迭代器 http://grepcode.com/file/repository.grepcode.com/java/root/jdk/openjdk/6-b14/java/util/Iterator.java
Basically speaking, both of them are very closely related to each other.
Consider Iterator to be an interface which helps us in traversing through a collection with the help of some undefined methods like hasNext(), next() and remove()
On the flip side, Iterable is another interface, which, if implemented by a class forces the class to be Iterable and is a target for For-Each construct.
It has only one method named iterator() which comes from Iterator interface itself.
When a collection is iterable, then it can be iterated using an iterator.
For understanding visit these:
ITERABLE: http://grepcode.com/file/repository.grepcode.com/java/root/jdk/openjdk/6-b14/java/lang/Iterable.java
ITERATOR http://grepcode.com/file/repository.grepcode.com/java/root/jdk/openjdk/6-b14/java/util/Iterator.java
Iterable
被引入用于 java 中的每个循环。Iterator
是管理Iterable
迭代的类。它维护当前迭代中所处的状态,并知道下一个元素是什么以及如何获取它。Iterable
were introduced to use in for each loop in javaIterator
is class that manages iteration over anIterable
. It maintains a state of where we are in the current iteration, and knows what the next element is and how to get it.除了 ColinD 和 Seeker回答。
简单来说,Iterable和Iterator都是Java的Collection Framework中提供的接口。
Iterable
如果一个类想要有一个 for-each 循环来迭代它的集合,那么它必须实现 Iterable 接口。但是,for-each 循环只能用于向前循环集合,并且您将无法修改此集合中的元素。但是,如果您想要的只是读取元素数据,那么这非常简单,并且由于 Java lambda 表达式,它通常是一个行。例如:
Iterator
此接口使您能够迭代集合,获取和删除其元素。每个集合类都提供一个 iterator() 方法,该方法将迭代器返回到集合的开头。与可迭代相比,此接口的优点在于,使用此接口您可以添加、修改或删除集合中的元素。但是,访问元素需要比可迭代元素多一点的代码。例如:
来源:
In addition to ColinD and Seeker answers.
In simple terms, Iterable and Iterator are both interfaces provided in Java's Collection Framework.
Iterable
A class has to implement the Iterable interface if it wants to have a for-each loop to iterate over its collection. However, the for-each loop can only be used to cycle through the collection in the forward direction and you won't be able to modify the elements in this collection. But, if all you want is to read the elements data, then it's very simple and thanks to Java lambda expression it's often one liner. For example:
Iterator
This interface enables you to iterate over a collection, obtaining and removing its elements. Each of the collection classes provides a iterator() method that returns an iterator to the start of the collection. The advantage of this interface over iterable is that with this interface you can add, modify or remove elements in a collection. But, accessing elements needs a little more code than iterable. For example:
Sources:
匿名类可以轻松地将
Iterator
转换为Iterable
,并允许您使用Iterable
语法(for 循环、forEach())。
示例:考虑一个
Iteratorit
抽象为函数
用法
An anonymous class easily converts an
Iterator
to anIterable
and lets you use theIterable
syntax (for loops,forEach()
).Example: consider an
Iterator<T> it
Abstracted in a function
Usage
大多数 Java 接口以 'able' 结尾。例如Iterable、Cloneable、Serialized、Runnable等。因此,Iterable是一个接口,有一个名为 iterator() 的抽象方法,该方法返回一个 Iterator 对象。
迭代器接口具有抽象方法“hasNext()”和“next()”。
一个明确的用途是 List 扩展 -> Collections Interface -> 扩展 Iterable
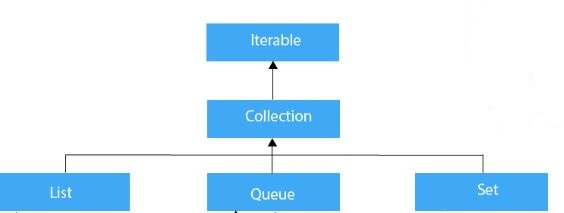
Most java interfaces ends with 'able'. examples are Iterable, Cloneable, Serializable, Runnable, etc. Therefore, Iterable is an interface that has an abstract method called iterator() which returns an Iterator object.
Iterator interface has abstract method 'hasNext()' and 'next()'.
A clear use is a List which extends->Collections Interface->extends Iterable
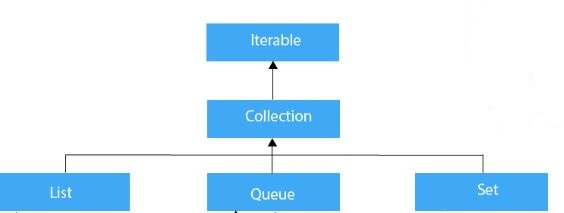